With C# 13, you can specify the ESC character much more concisely as shown in the following code snippet:
char esc="\e";
Implicit index access
With C# 13, the implicit “from the end” index operator ^
can now be used in object initializers. You can use ^
to specify a position in a collection that is relative to the end of the collection.
For example, consider the following class.
class InitializerDemo
{
public int[] integers { get; set; } = new int[5];
}
You can now use the following piece of code in C# 13 to take advantage of the index operator.
var arr = new InitializerDemo
{
integers =
{
[0] = 100,
[^1] = 1000
}
};
When you execute the above program, arr.Integers[0]
will have the value 100 while arr.Integers[4]
will have the value 1000. You can use the following line of code to display the values of the integer array at the console.
Console.WriteLine("The value of arr.Integers[0] is {0} and arr.Integers[4] is {1}", arr.Integers[0], arr.Integers[4]);
Figure 2 shows the output at the console when the code is executed.
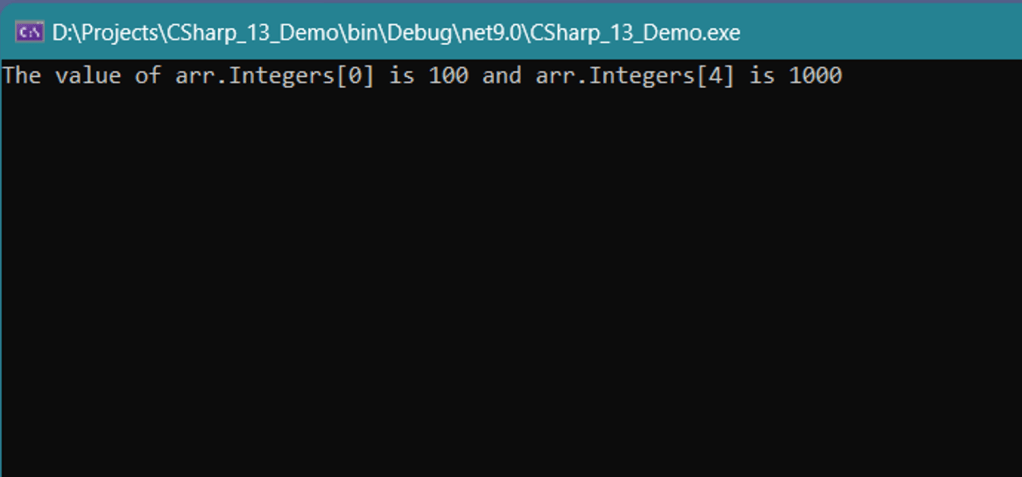
Figure 2. The implicit “from the end” index operator ^ in action.
IDG
TargetFramework .NET 9
Note that you will need to have .NET 9 installed in your computer to work with C# 13. If you want to change your existing projects to use C# 13, you will need to set the TargetFramework
to .NET 9 as shown in the code snippet given below.
The new features and enhancements in C# 13 outlined in this article will give you more flexibility and help you write cleaner, more maintainable C# code. To explore the new features of C# 13, you should download the latest preview of Visual Studio 2022 with .NET 9. You can learn more about the new features in C# 13 here and here.